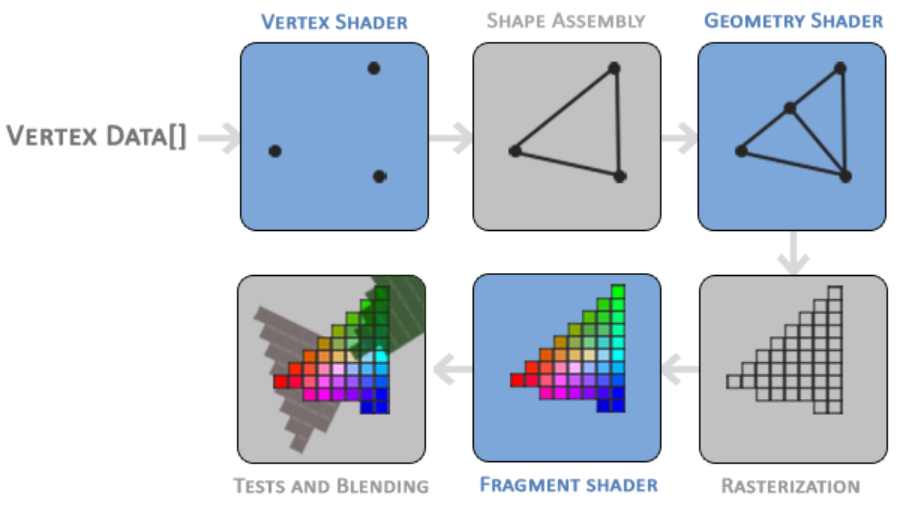
Create a program that reads in and renders a triangle mesh (of type .obj) to an image via software rasterization. You may use existing resources to load in the mesh and to write out an image. You must write your own rasterizer. In general, the required steps for the program are:
Read in triangles.
Compute colors per vertex.
Convert triangles to image coordinates.
Rasterize each triangle using barycentric coordinates for linear interpolations and in-triangle tests.
Write interpolated color values per pixel using a z-buffer test to resolve depth.

Every triplet of three consecutive vertices in this array forms a triangle. In other words, every nine elements form the (x,y,z) coordinates of the three vertices of a triangle. (For now, you can ignore the normal and the texture coords.)
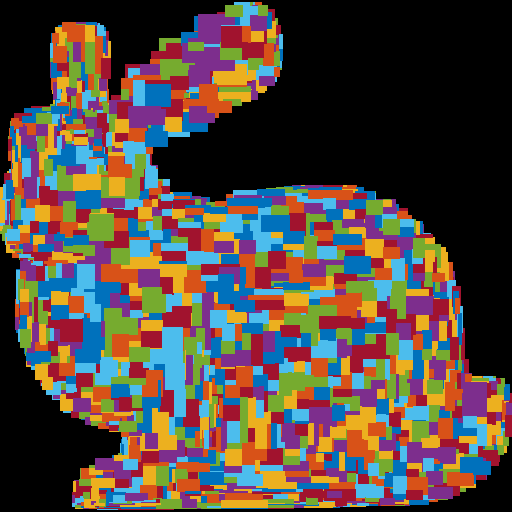
Drawing Bounding Boxes
Write code to convert each 3D coordinates into 2D image coordinates. Assume the camera is at the origin looking down the negative z axis. Make sure the object completely fills the image without any distortion.
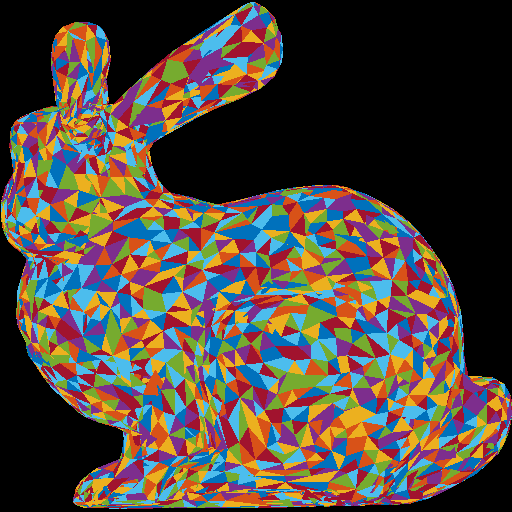
Drawing Triangles
need a small “epsilon” when checking the barycentric coordinates to deal with floating point errors. In other words, rather than checking to see if a barycentric coord is greater than exactly zero, check to see if it is greater than a small negative value.
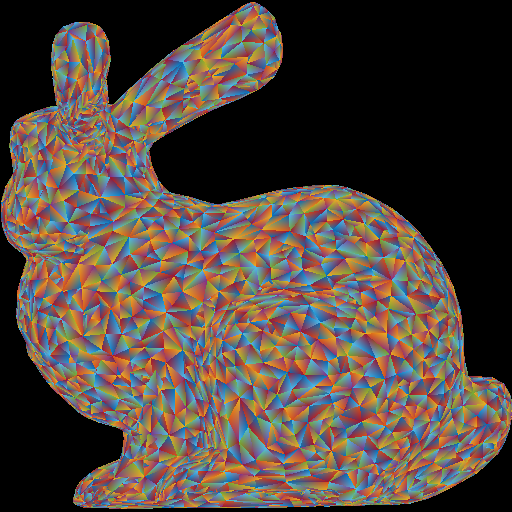
Interpolating Per-Vertex Colors
Barycentric coordination
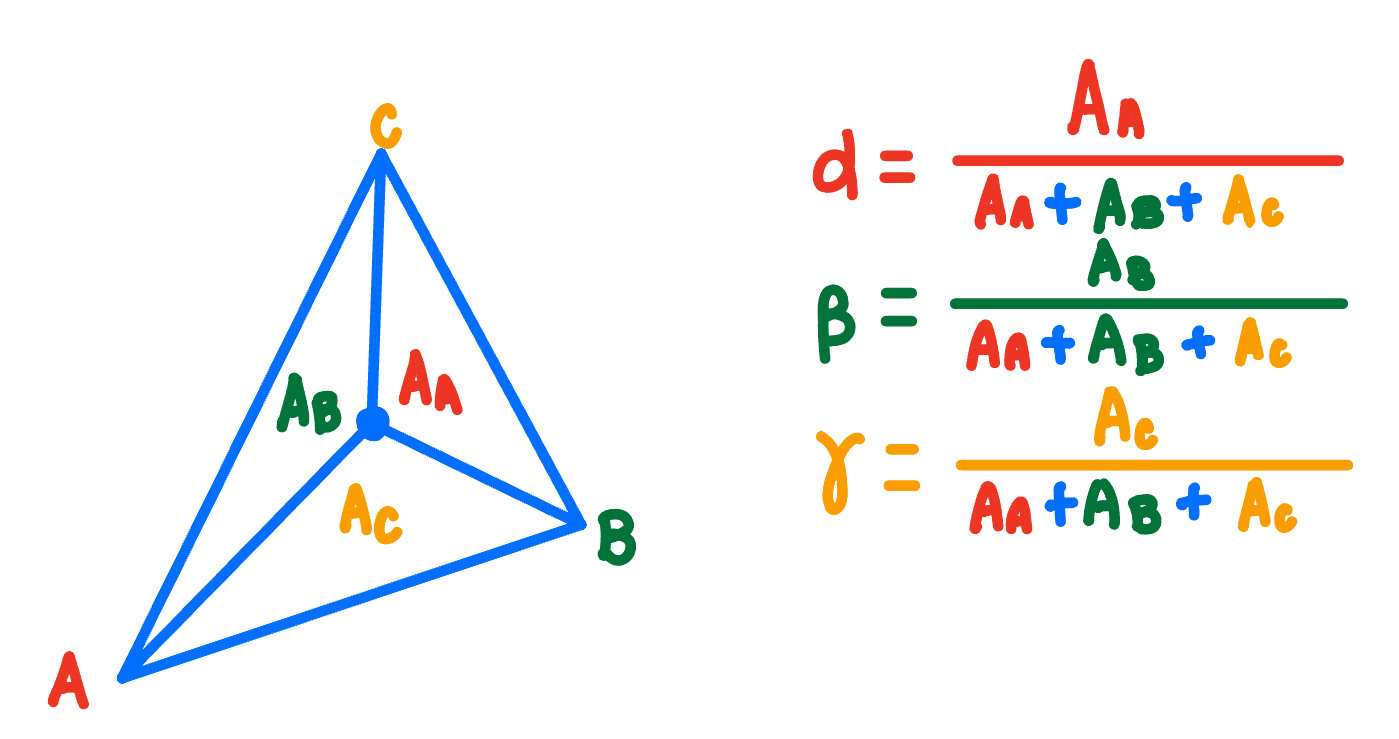
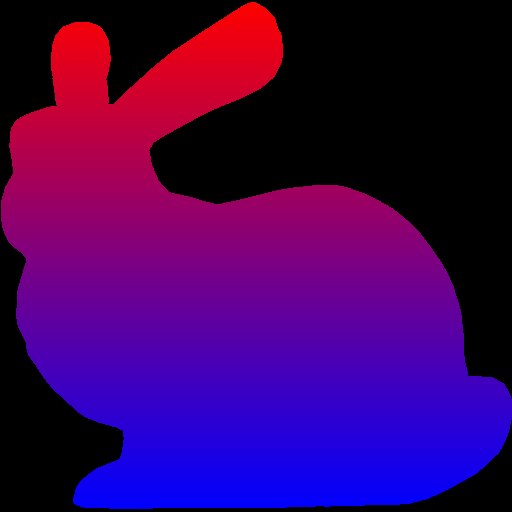
Vertical Color
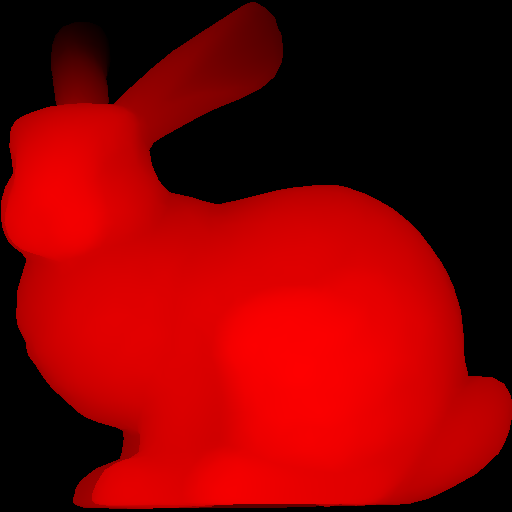
Z-Buffering
create a data structure to support z-buffer tests. Your z-buffer should be a separate buffer from your image pixel buffer, and it should be the same size as your pixel buffer. The z-buffer contains the z-coordinate of each pixel, which is interpolated from the z-coordinates of the three vertices using the pixel’s barycentric coordinates.
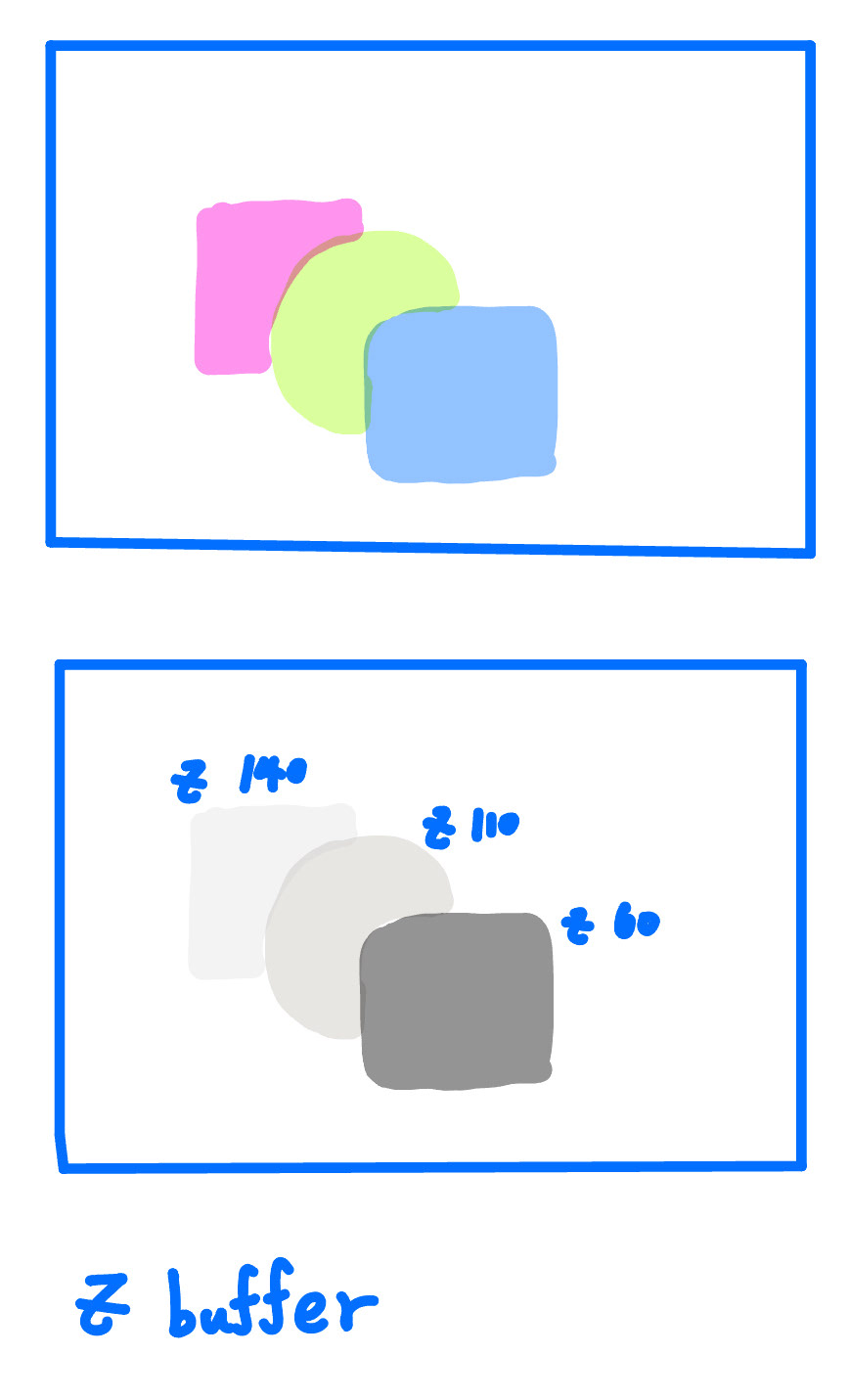
The Z-buffer ensures correct 3D rendering by storing the closest depth for each pixel, updating only when a fragment is closer to the camera. This prevents visual overlaps and handles occlusion efficiently.
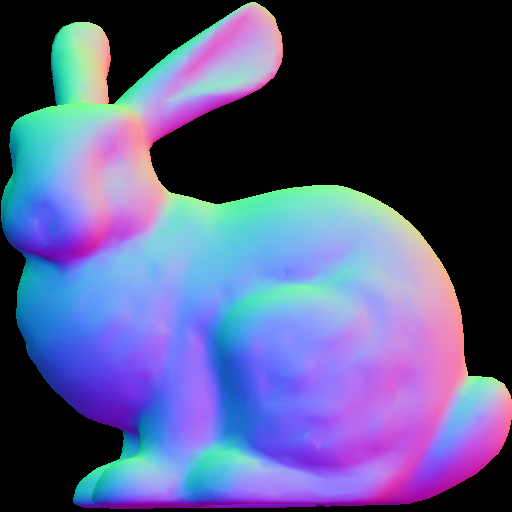
Normal Coloring
These are stored in the norBuf variable alongside the posBuf variable that is already being used. Store this “normal” information (a 3D vector) in each vertex. Then, when coloring the pixels, interpolate the normals of the three vertices of the triangle to compute the normal of the pixel.
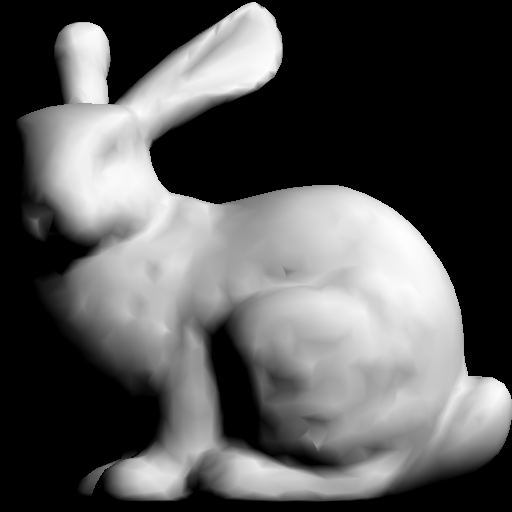
Simple Lighting
Then, when coloring the pixels, interpolate the normals of the three vertices of the triangle to compute the normal of the pixel.
Rotation Matrix
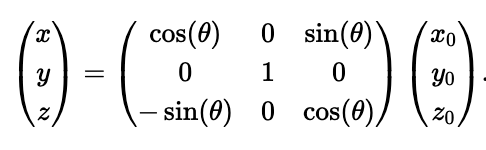
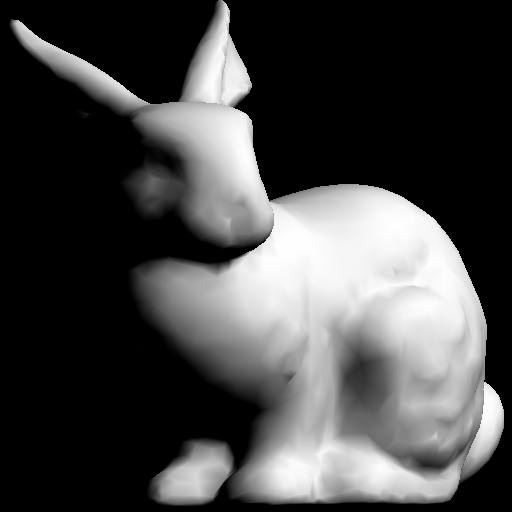
Rotate
Apply a rotation of π/4 radians (45 degrees) about the Y axis. The rotation should be applied immediately after loading the vertices, before fitting to the window. This ensures that the rotated object fits the window exactly as before. Notice that the teapot appears bigger than before, since the rotated teapot takes less horizontal space in window coordinates.
Transform both the vertex position and the normal using the equation below: